Prev Next
Three things need to be done to make a java program work. They are,
1. Create,
2. Compile, and
3. Run
- A source file is to be created with .java extension.
- The source file is then compiled by the java compiler which yields a class file with. class extension
- This class file is run by the JVM and yields the result.
- A class file, when it is created in one platform can run and give the same result in another platform. For example, a class file generated in a windows environment can run and give the same result in Linux environment. That is why, java is platform independent.
Java Hello World Example Program: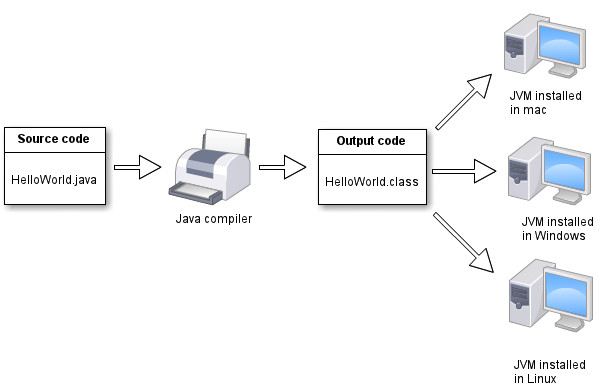
HelloWorld.java
1 2 3 4 5 |
class HelloWorld { public static void main(String[] args){ System.out.println("Hello World"); } } |
Output:
C:\> javac HelloWorld.java C:\> java HelloWorld Hello World |
1. Creating a source file:
Type the above program in a notepad and save the file with the name that should match exactly with the class name and with .java extension. That is HelloWorld.java
2. Compiling the source file:
Open command prompt and go to the directory where HelloWorld.java is located and type the command
javac HelloWorld.java |
The program will be compiled by javac compiler and HelloWorld.class file is created and ready to be executed.
3. Run the program:
Then, type the command.
java HelloWorld |
This command will execute the class file and displays the output ‘Hello World’.
Pre-requisite:
Need to install jdk latest version and JRE. Otherwise, you can try using an IDE like RAD or Eclipse to create, compile and execute Java programs.
A simple/Basic Java Program with explanation:
1 2 3 4 5 |
public class HelloWorld{ public static void main(String[]args){ System.out.print("Hello World"); // prints Hello World } } |
- A basic java program would contain a class and a main method with some statements inside it.
- Everything in java must be inside a class. The program is saved with .java extension.
- The name of the file must be same as that of the class name. Here it is saved as ‘HelloWorld.java’.
Note: Java is case sensitive and hence make sure that the class name and the filename matches.
Compiling the source file:
- To compile the java source file, open the command prompt and navigate to the directory where the source file exists.
- The following command is typed to compile the program. (Here it is assumed that the java execution path variable is set)
javac HelloWorld.java |
- Now, Java compiler generates a file named ‘HelloWorld.class’ which would contain the bytecode instructions of the source file.
- Bytecode is a format which the JVM understands.
- The Java Virtual Machine (JVM) is an abstraction layer between a Java application and the underlying platform.
- As the name implies, the JVM acts as a “virtual” machine or processor which interprets the bytecode.
Running the program:
The class file generated is executed by the following command.
java HelloWorld |
The class name alone is passed to the java interpreter, which identifies the class file and interprets the bytecode information.
[]
Understanding the first java program:
- In Java, class is the basic unit of encapsulation which contains all the code.
- A class is defined by the keyword ‘class’ followed by the class name. i.e as
public class HelloWorld{
The keyword ‘public’ is access specifier. Java Access Specifiers (also known as Visibility Specifiers ) regulate access to classes, fields and methods in Java. There are 4 types of access specifiers.
- public – can be accessed outside of the class in which it is declared.
- private – can be accessed only within the class in which it is declared.
- protected – can be accessed within the class, and in the subclasses of it.
- default – if no modifier is specified, it is considered to have default access. i.e. it is accessed anywhere within the package where the java class is defined.
A Java Application begins its execution from the main method.
public static void main(String[] args){
- The keyword ‘public’ represents that the main method could be called outside of the class.
- The keyword ‘static’ helps the main method to be called before an object of the class is created.
- The keyword ‘void’ indicates that the method returns nothing.
- We may also change the return type of the main method if required.
The string array ‘args’ is used to pass any command line arguments while running the program.
System.out.print(“Hello World”);
The above line is a statement. Every statement ends with a semicolon. The print() method is used to display the string specified. System.out refers to the object which represents the console. System is a class defined in java.lang package. This package is imported by the JVM implicitly. Packages are explained in the end. ‘out’ is an instance of the PrintStream class.
The braces {…} marks the beginning and the end of the class, method and block.
// prints Hello World
The above line represents a comment. Comments are used for the purpose of the user and it is not executed by the program. There are 3 kinds of comments in java.
- Single line comment – // text
Used for single line comments. Everything after the ‘//’ is ignored
- Multi line comment -/*comment1
*continued
*/
Used for multiple line comments. Begins with /* and ends with */
- Documentation comment (Doc comment) –
/**
*
*/
Doc comments are ignored by the compiler, but they can be extracted and automatically turned into HTML documentation by the javadoc tool.
Example : @author, @version, @exception, @param, @return are some of the doc comments each having its own meaning.
Package:
A package is a namespace that organizes a set of related classes and interfaces. Some of the existing packages present in java are java.io, java.util, java.awt etc. Users can also create their own package and categorize classes and interfaces. Packages can be created using the keyword package followed by the package name.
Syntax for package creation:
1 |
package package_name; |
Import statements:
- Import statement provides the exact location of a particular system/user defined package whose classes and members are to be used in the current program.
- Package and import statements must be specified in the beginning of the program.
Example:
1 2 3 4 5 6 7 8 |
package MyApp; //class A is defined under the package MyApp import java.io.*; //importing the io package class A{ …… } |
The import statement in the above class, would load all the classes and interfaces present inside the java.io package.